Introduction
Map Generator
is used for creating statistic maps.
Geographic statistic map ("map") is intended for graphic representation of
certain value in map with color background, where backgroud color in given position
represents intensity of the value with regard to chosen color scale.
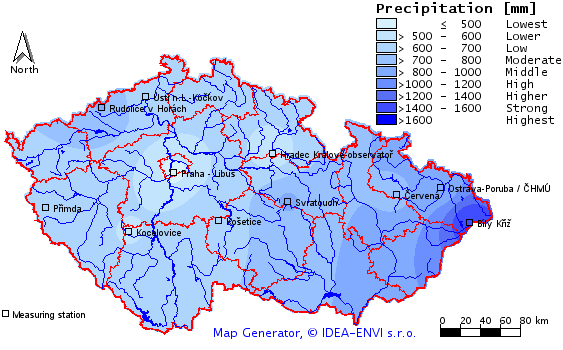
Maps contain color background, additional information (borders, cities…) and a legend with color scale and value ranges, that represent particular background colors.
Basic information
Coordinate System
Map Generator uses internally Cartesian coordinate system . The system is unitless - it does not matter, which units are used. It required to define the relation between coordinate system unit and pixel on bitmap (map scale).
For creating maps of the Czech Republic we use Gauss-Krüger S-42 coordinate system. Also it is possible to use any other coordinate system that can be transformed to two dimensional cartesian coordinates, e.g. WGS 84.
Quantity
Quantity is type of values, displayed on map – it can be any statistical value, such as unemployment rate (percentage) , measured concentration of substance (air quality), temperature, amount of precipitation (or other hydrology data) etc.
Input data
Input data are primary values, which create a map after processing. If we want to create a map, we need input data of our quantity. Every datum of input data must have the following information:
- Geographic position of place, where the value was obtained or measured
- Name of place, where the value was obtained – for example name of the measuring station
- The obtained or measured value
Input data may be loaded into program from XML files, or using extensions is possible to connect program to any external data source.
Color scale
Color scale is a table for conversion of value range to color.
Matrix
Matrix, a.k.a grid, is a two-dimensional value array that is basic unit of data processing in Map Generator. For our purposes we divide matrixes to:
- Input matrix contains prepared values, such as terrain altitude, population density etc. It can be loaded from a file or database
- Computed matrix contains values that are the result of interpolation of input values
- Result matrix contains values that are the result of combination of values in other matrixes
All the matrixes that come together into map generation process must have equal sizes (cell size and cell counts in both axis) and geographic position of the left bottom corner.
Data processing
While map generation, input values are processed using various algorithms.
Interpolation
Interpolation is used to infer values into cells of computed matrix. Those values depend on input values. Every computed matrix must have associated interpolation algorithm. At this time IDW algorithm is implemented.
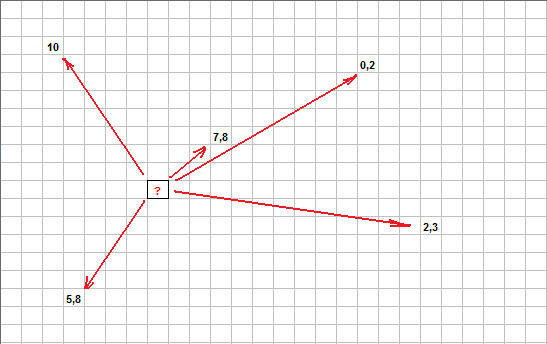
On image you can see matrix that has input values in some cells. Other cells are void - their values are unknown, because input values at those places are not obtained (or measured).
For each of the void cell weighted average is computed from input values using IDW, where weight is the distance between computed cell center and place where input value was given. Nearer input values affect result value more, further less. This IDW explanation is pretty simple - reality is more difficult.
Modification
Modification traverses cells in one or more matrixes, performs computation using particular algorithm and stores result into original or newly created matrix.
This allows to modify matrix values -perform corrections, combine their values etc.
Rendering
Rendering get matrix, that contain final values and create map background. Every cell value is converted into color and stored into particular pixel in map.
In the next phase, color background is repainted with vector shapes – borders, legend, measuring stations etc.
The result is (by default) a PNG image. Other types
(BMP, GIF, JPG) are also supported. Using Java API it is also possible to
join more generation processes.
Data processing control
The process of map generating can be very flexible. Some maps are created from more matrixes, others only from one. Also, other algorithms ale optional and fully configurable.
There are two ways to take control over data processing:
- Using XML file (the Runset)
- Using API
XML Runset
Runset takes control over data processing in Map Generator from loading input values, interpolations into matrixes, combinations, modifications, rendering, to storing the final map into a file or database.
Runset is XML file, where particular XML elements represent objects in Map Generator. By editing Runset it is possible to change Map Generator behavior and tune the result by our requirements.
Java API
MapGenerator is created on Java SE platform.
Using Java (alternatively Groovy) language it is possible to control map generating. You can create your own Java project in
Eclipse IDE (for example) and
incorporate MapGenerator.jar
into it. By calling API in MapGenerator classes it is possible to control map generating
process.
Extending program
While developing of Map Generator, we focused on flexibility and extensibility. MapGenerator is able to generate statistical maps from every area and it's abilities can be extended. It is possible to develop own combination and modification algorithms, input data sources, map writers, graphic objects (shapes) etc.
This extensibility in practice is provided by ability of Java to load classes at runtime using classloader .
Most of objects in XML Runset have attribute class
, that contain Java class-name, that provide required functionality.
In the case that user of Map Generator found existing Interpolation algorithm as unsatisfactory, it is possible to program own one and in Runset replace attribute
class content class="idea.map.interpolator.IDWInterpolator"
by name of your own class.
Under one condition that the user class must be derived from class idea.map.interpolator.AbstractInterpolator
,
to comply contract between user class and rest of program.
ISKO Map Generator
Example of Map Generator extension is project ISKO Map Generator that we developed for CHMU. This program generates air pollution maps from ISKO 2 database.
Maps are hourly generated from values in DB ISKO2 and stored back into this database. Two computing servers cooperative by divide this work, in case that one fails, the other machine computes maps with greater priority in all available time.
For presentation is used JSF application IskoPollutionMapView on application server.
MapGenerator SDK
This SDK is for users with knowledge of Java language. It contains API documentation of Map Generator classes and
Eclipse project MapGeneratorExtension
- set of user classes samples. This source code can be used
as a starting point to learn, how to extend Map Generator by own functionality.
UserModificator.java
UserModificatorThread.java |
Sample of user modificator algorithm - modifies one matrix |
UserCombinator.java
UserCombinationThread.java |
Sample of user modificator algorithm - combines three matrixers into another one |
UserInterpolator.java
UserInterpolatingThread.java |
Skeleton of user interpolation algorithm |
MapWriterToFtp.java | Map writer, uploads map to FTP server |
DataValuesFromPgSQL.java | Loads input data from PostgreSQL table |
ListColorScaleFromPgSQL.java | Loads color scale from PostgreSQL table |
TextWithShadow.java | Sample of user defined simple shape - shadowed text |
Hamburg.java | Sample of user defined complex shape - city Hamburg |
RotatedRectangles.java | Sample of user shape, demonstrates rotation of cartesian positions |
SegmentedPolygon.java | Sample of user shape, demonstrates rotation of polar positions |
AdministrativeAreaFromKML.java | Sample of user shape, dynamicaly created from KML file. Uses Java API for KML - JAK |
RunSet1.xml | Sample of Runset, shows how to use sample classes DataValuesFromPgSQL and ListColorScaleFromPgSQL |
RunSet2.xml | Sample of Runset, shows how to use custom shapes |
HamburgPM10.xml | Sample of Runset, shows how to create simple pollution map |
CzechRepublicFromKML.xml | Sample of Runset, shows how to use AdministrativeAreaFromKML, create map of Czech Republic from KML files |
GermanyFromKML.xml | Sample of Runset, shows how to use AdministrativeAreaFromKML, create map of Germany from KML files |
MapGeneratorExtension.java | Extends class MapGenerator on connection to PostgreSQL database |
Demo1.java | Does this same job as RunSet1.xml but using Java API |
Demo2.java | Does this same job as RunSet2.xml but using Java API |
RunMapGeneratorExt.java | Application entry point |
Another sample project MapGeneratorWeatherSample
- utilizes Groovy language for creating
simple weather map, doing three steps:
- Loads airports.xml file from input directory
- Obtains weather data for airports from Internet Weather source
- Visualises weather data using Map Generator
airports.xml | Airport codes, names and geo positions are stored here |
Airport.groovy | Contains airport data from XML and weather info |
Weather.groovy | Contains weather data - decoded METAR |
DataValueWithMetar.groovy | Contains air pressure value with raw METAR included |
WeatherDataValues.groovy | Data values to visualize on the map - use airport air pressure (QNH) and includes raw METAR for tooltips |
MyHtmlImageMapItems.groovy | Adds raw METAR into standard HTML tooltips |
Main.groovy | Entry point, create map |
Do you Want to Create your Own Maps ?
You can create own maps, using Map Generator. Before trying it, you need following:
- Be familiar with XML - you must be able to create and modify Runsets
- Understand, how Map Generator works - read the User Guide
- Be familiar with GIS - you must understand how coordinate systems work and more
- Have input (measured) data, and be able to convert it to XML format
- Have GIS data for area, where you want to create a map, e.g. city or country borders, etc.
- Acquire any variant of Map Generator
- For coding your own Map Generator extensions, you need the knowledge of Java or Groovy language
Or you may leave this job to us ...
License
Program Map Generator is available in following variants:
- Free edition - free for non-commercial using or testing. Allows control using XML Runset.
- Standard edition - allows to create maps for internal company/organization use only. Contains MapGenerator SDK for creating your own extensions.
- Professional edition - allows to sell created solution to another organization.
Professional and Enterprise editions contain MapGenerator SDK. It allows extending and control program using Java API.
If you are interested in acquiring any variant, contact as.
Purchase
Item | Price | Description |
---|---|---|
Standard edition | 900 € | License per one developer, with Annual Technical Support |
Professional edition | 3000 € | License per one developer, with Annual Technical Support |
Annual Technical Support | 300 € | License per one developer, email based support |
We can also develop custom Map Generator extension for you or prepare geographic data, create Runsets etc. Price of this work depends on the amount of time, and it must be specified individually.
If you are interested in extending Map Generator (connect to own database for example), and don't have required skills of Java programming - contact.
If you want to create your own map and need GIS data - contact .
Documentation
Download User Guide.
Maps gallery
Map of concentration SO2
SO2 concentration, hourly average, generated from ISKO2 database.
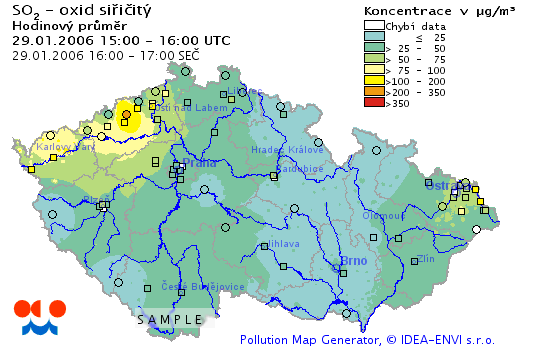
Dust above central Europe
On the 24th March 2007, many countries of central Europe were covered by dust, falling with the rain. The origin of this dust was from eastern Ukraine.
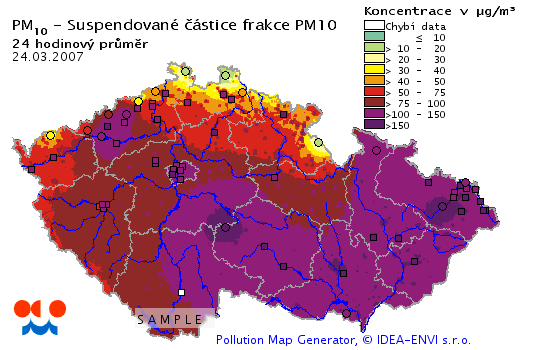
Data source - CHMI
Temperature Inversion Impact Air Quality
Czech TV, channel CT24 - World Weather 19.1.2009
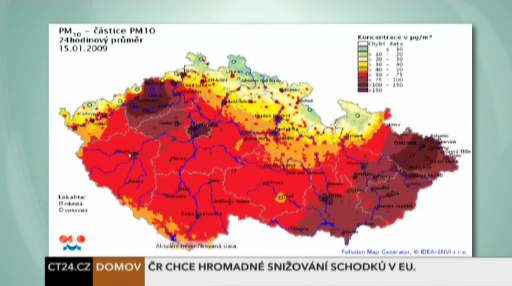
Impact of winter temperature inversion to Air Quality in the Czech Republic.